Algorithms and data structures often feel like abstract, academic exercises when you first encounter them. Many of us in university wondered, Will I ever use this in real life? Fast forward to the working world, and the answer is often a resounding yes. Here’s a story of how I used Breadth-First Search (BFS), a classic algorithm, to solve a seemingly impossible problem for a restaurant inventory system.
The Restaurant Dilemma
Imagine you’re designing a system for a restaurant chain. The system needs to handle recipes, track ingredient usage, and calculate costs for menu items. Sounds straightforward, right? Let’s complicate it.
Consider a dish like Lasagna. To make lasagna, you need:
- Pasta Sheets
- Bolognese Sauce
- Cheese
But here’s the twist:
Bolognese Sauce is itself a recipe that requires:
- Ground Meat
- Tomatoes
- Spices
Every ingredient comes from an inventory with details like:
- Unit of Measurement (UOM): Grams, Liters, etc.
- Cost per Unit: Calculated based on the last purchase price or weighted average.
Now, when a customer orders lasagna, the system needs to:
- Break down the recipe into its components, all the way to the raw ingredients.
- Calculate the exact quantities of each ingredient used.
- Determine the total cost of making one lasagna.
- Deduct the ingredients from the inventory.
This process is manageable for simple, two-level recipes like Lasagna → Bolognese Sauce → Ground Meat. But what happens when we introduce deeper nesting?
A Deeper Nested Recipe
Consider a Vegetarian Pizza as an example of a more nested recipe:
- Vegetarian Pizza– Dough– Flour– Water– Yeast
- Tomato Sauce– Tomatoes– Olive Oil– Herbs
- Cheese
- Toppings– Grilled Vegetables— Zucchini— Bell Peppers— Eggplant
This structure involves:
- A top-level recipe (Vegetarian Pizza).
- Mid-level recipes (Dough, Tomato Sauce, Toppings).
- Raw ingredients at the leaf level (Flour, Water, Tomatoes, etc.).
When a customer orders a pizza, the system must calculate the total cost by traversing all five levels and summing up the costs of every ingredient.
A Simple Calculation Example
Let’s use the Vegetarian Pizza example to calculate the total cost step by step.
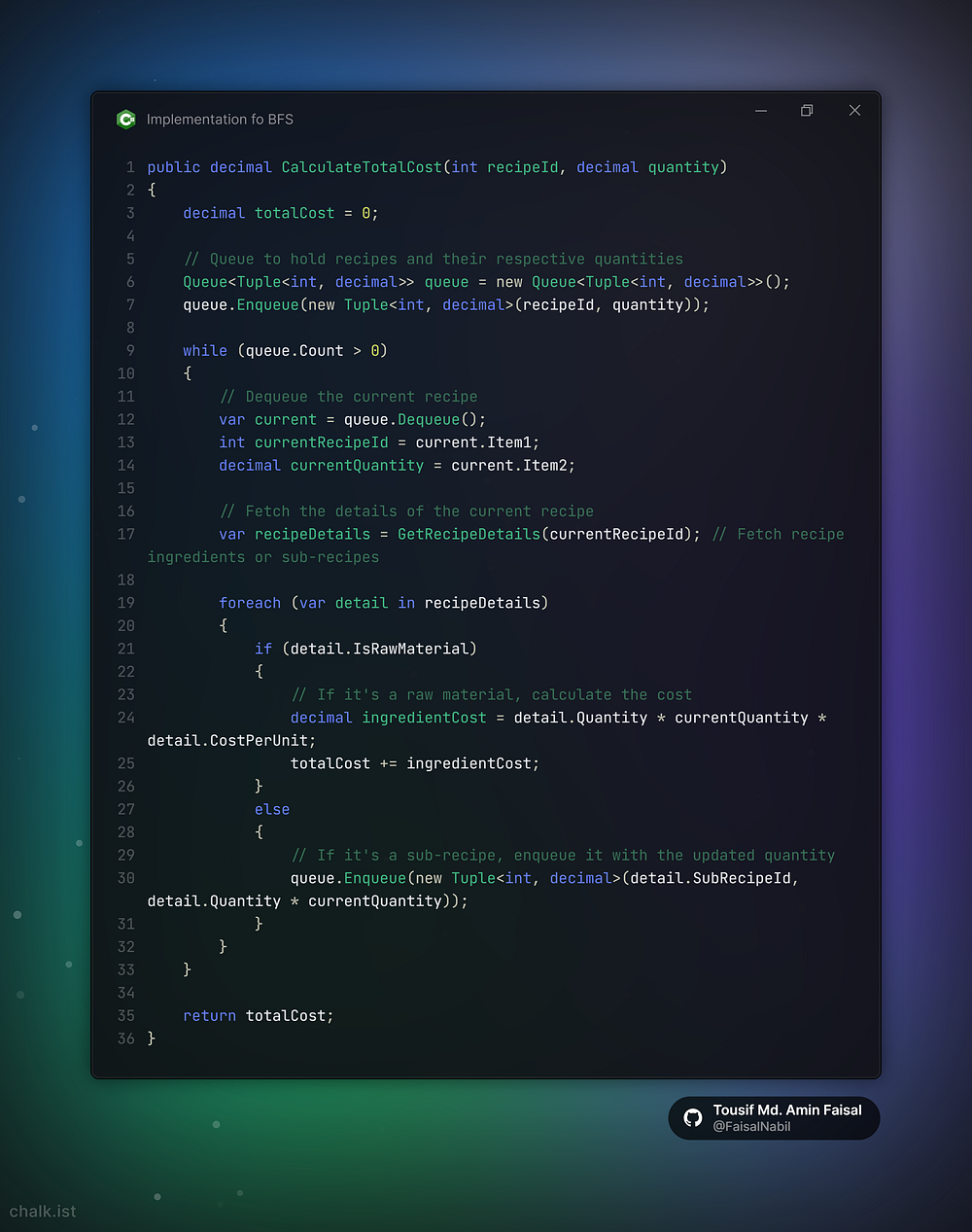
Ingredients and Costs
- Vegetarian Pizza requires:- Dough— 500g Flour (cost per gram = $0.01)— 300ml Water (cost per ml = $0.002)— 10g Yeast (cost per gram = $0.05)- Tomato Sauce— 200g Tomatoes (cost per gram = $0.03)— 50ml Olive Oil (cost per ml = $0.1)— 5g Herbs (cost per gram = $0.2)
- Cheese— 200g Cheese (cost per gram = $0.05)
- Toppings-100g Grilled Vegetables— 50g Zucchini (cost per gram = $0.02)— 30g Bell Peppers (cost per gram = $0.03)— 20g Eggplant (cost per gram = $0.025)
Step-by-Step Calculation
Dough Cost:
- Flour: 500g × $0.01 = $5
- Water: 300ml × $0.002 = $0.60
- Yeast: 10g × $0.05 = $0.50Total Dough Cost = $6.10
Tomato Sauce Cost:
- Tomatoes: 200g × $0.03 = $6
- Olive Oil: 50ml × $0.1 = $5
- Herbs: 5g × $0.2 = $1Total Tomato Sauce Cost = $12
Cheese Cost:
- 200g × $0.05 = $10
Toppings Cost:
- Zucchini: 50g × $0.02 = $1
- Bell Peppers: 30g × $0.03 = $0.90
- Eggplant: 20g × $0.025 = $0.50Total Toppings Cost = $2.40
Vegetarian Pizza Cost:
- Dough: $6.10
- Tomato Sauce: $12
- Cheese: $10
- Toppings: $2.40Total = $30.50
Cost Relationships in Pricing
Once the total cost is calculated, it’s important to set prices effectively using markup cost, portion cost, and selling price. These relationships depend on business logic, but they can be defined as follows:
Cost Calculation Logic
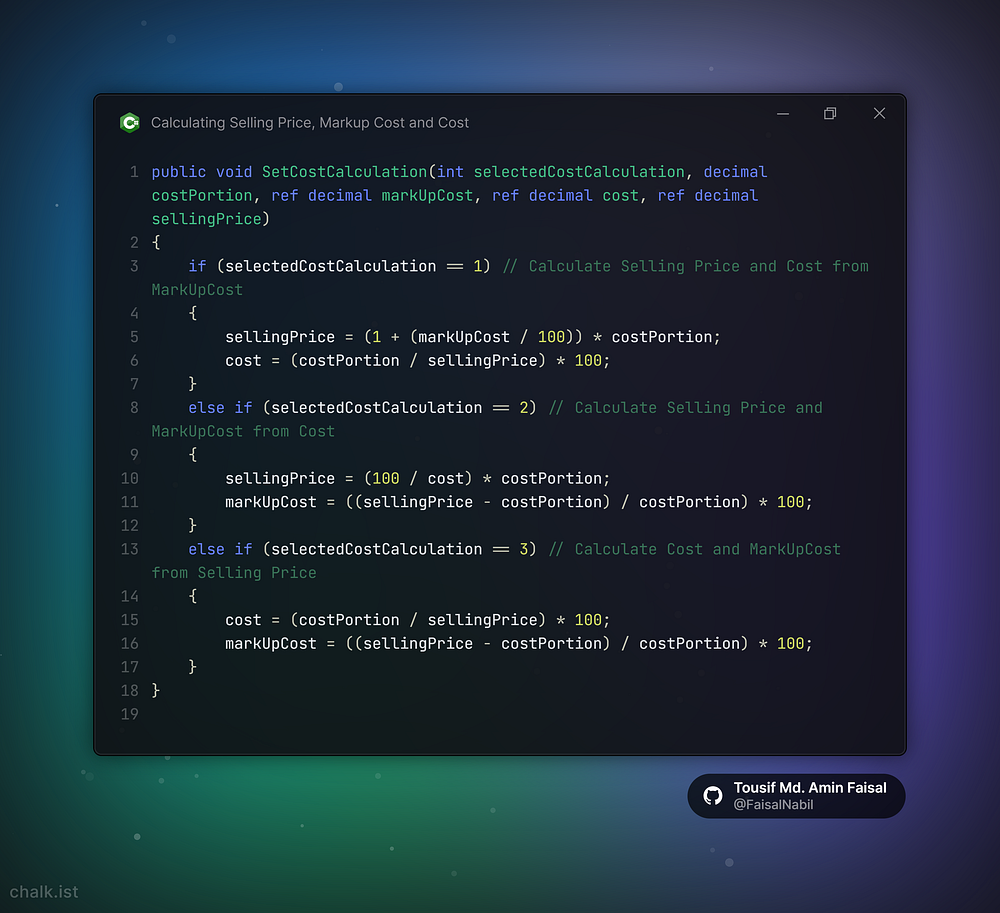
Explanation
- Option 1: If you know the markup percentage, calculate the selling price and cost.
- Option 2: If you know the cost percentage, calculate the selling price and markup percentage.
- Option 3: If you know the selling price, calculate the cost and markup percentage.
Bringing It All Together
With BFS to traverse recipes and a clear cost calculation logic, the restaurant system now:
- Handles recipes with any level of nesting.
- Dynamically calculates costs and prices for dishes.
- Automates inventory management and updates.
Comments
Post a Comment